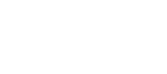
Pillow
オンラインドキュメント
https://pypi.org/project/Pillow/
https://pillow.readthedocs.io/en/latest/installation.html
https://pillow.readthedocs.io/en/latest/handbook/index.html
インストール
pip install pillow |
1 |
CentOS7環境
# pip3.6 install pillow |
1 |
Fedora33環境
# dnf install python3-pillow |
1 |
使い方
ファイル形式の変換
from PIL import Image |
im = Image.open("a1.jpg") |
im.save("a1.png") |
1 |
2 |
3 |
4 |
線の描画
from PIL import Image, ImageDraw |
im = Image.new('RGB', (256,100), (255, 255, 255)) |
d = ImageDraw.Draw(im) |
for cnt in range(128) : |
d.line([(0+cnt*2,0),(0+cnt*2,49)], fill=(0,128+cnt,0), width=2) |
d.line([(0+cnt*2,50),(0+cnt*2,99)], fill=(0,255-cnt,0), width=2) |
im.save("b5.bmp") |
1 |
2 |
3 |
4 |
5 |
6 |
7 |
8 |
9 |
10 |
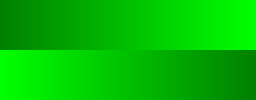
透明PNG、多角形、テキストの描画
from PIL import Image, ImageDraw, ImageFont |
im = Image.new('RGBA', (240, 40), (0, 0, 0, 0)) |
d = ImageDraw.Draw(im) |
font1 = ImageFont.truetype("fonts/ipagp.ttf", 24) |
d.polygon([(39,0),(0,19),(0,39),(199,39),(239,19),(239,0),(39,0)], fill=(255,215,0,255)) |
d.line([(39,0),(0,19),(0,39),(199,39),(239,19),(239,0),(39,0)], fill=(128,128,128,255), width=3, joint="curve") |
t_size = d.textsize("Play", font=font1) |
x = int( (240 - t_size[0])/2 ) |
y = int( (40 - t_size[1])/2 ) |
d.text((x,y), "Play", font=font1, fill=(0,0,0,255)) |
im.save("poligon_gold-olive.png") |
1 |
2 |
3 |
4 |
5 |
6 |
7 |
8 |
9 |
10 |
11 |
12 |
13 |
14 |
15 |
16 |
