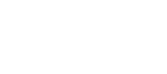
tornado-jinja2
オンラインドキュメント
https://pypi.org/project/tornado-jinja2/
インストール
pip install tornado-jinja2 |
1 |
使い方
helloアプリケーションの作成
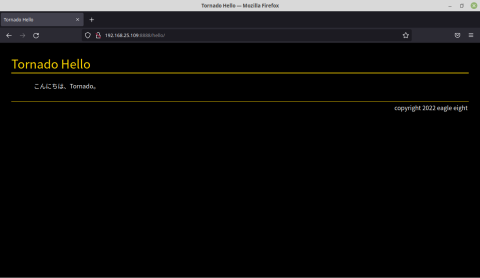
以下の内容で app_hello.py を作成する。
from tornado.ioloop import IOLoop |
import tornado.web |
from tornado_jinja2 import Jinja2Loader |
import jinja2 |
jinja2_env = jinja2.Environment(loader=jinja2.FileSystemLoader('templates/'), autoescape=False) |
jinja2_loader = Jinja2Loader(jinja2_env) |
settings = dict(template_loader=jinja2_loader) |
class hello(tornado.web.RequestHandler): |
def get(self): |
template = jinja2_env.get_template("index.html") |
text1 = '<h1>Tornado Hello</h1>\n' |
text1 += '<div class="result"><p>Hello,</p>\n<p>こんにちは,</p>\n<p>Bonjour bonjour,</p>\n<p>Merhaba,</p>\n<p>سلام,</p>\n<p>สวัสดี,</p>\n</div>' |
self.write(template.render(title="Tornado Hello", render_text=text1)) |
def make_app(): |
return tornado.web.Application([ |
(r"/hello/", hello), |
], |
autoreload=True |
) |
def main(): |
app = make_app() |
app.listen(8888) |
IOLoop.current().start() |
if __name__ == '__main__': |
main() |
1 |
2 |
3 |
4 |
5 |
6 |
7 |
8 |
9 |
10 |
11 |
12 |
13 |
14 |
15 |
16 |
17 |
18 |
19 |
20 |
21 |
22 |
23 |
24 |
25 |
26 |
27 |
28 |
29 |
30 |
31 |
以下の内容で templates/layout.html を作成する。
<!DOCTYPE html> |
<html lang="ja"> |
<head> |
<meta charset="UTF-8"> |
<title>{{title}}</title> |
<style> |
body { background-color: black; color: white; margin: 30px; } |
h1 { color: gold; border-bottom: double 3px; font-weight: normal; } |
h2 { color: navajowhite; border-bottom: solid 2px; border-left: solid 20px; padding: 3px; font-weight: normal; } |
h3 { border-bottom: solid 1px white; padding: 3px; font-weight: normal; } |
h4 { border-top: solid 1px gold; padding: 3px; margin-top: 30px; font-weight: normal; } |
h4 p { padding: 0px; margin: 0px; } |
.left { text-align: left; } |
.right { text-align: right; } |
.result { margin-top: 10px; margin-bottom: 10px; margin-left: 60px; margin-right: 60px; } |
</style> |
</head> |
<body> |
{% block body %}{% endblock %} |
</body> |
</html> |
1 |
2 |
3 |
4 |
5 |
6 |
7 |
8 |
9 |
10 |
11 |
12 |
13 |
14 |
15 |
16 |
17 |
18 |
19 |
20 |
21 |
以下の内容で templates/index.html を作成する。
{% extends "layout.html" %} |
{% block body %} |
{{render_text}} |
<footer><h4><p class="right">copyright 2022 eagle eight</p></h4></footer> |
{% endblock %} |
1 |
2 |
3 |
4 |
5 |
コマンドプロンプトで python app_hello.py を実行する。
http://localhost:8888/hello/ にアクセスして動作を確認する。
Firebied権限付与SQL作成アプリケーションの作成
Firebiedデータベースで、システム管理権限を持たないユーザに対し、ユーザ定義のテーブル、ビュー、シーケンス、プロシージャへのアクセス権限を付与するSQLを作成するアプリケーションです。
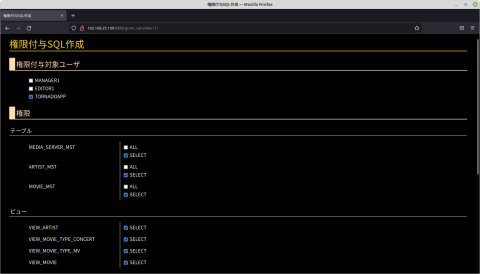
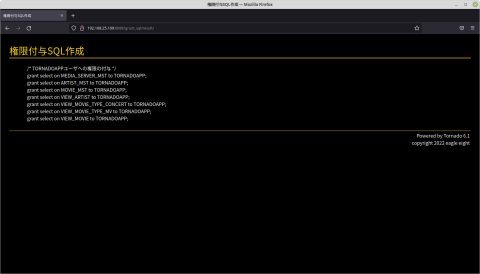
以下の内容で app_grant_sql.py を作成する。
from tornado.ioloop import IOLoop |
import tornado.web |
from tornado_jinja2 import Jinja2Loader |
import jinja2 |
import fdb |
import traceback |
jinja2_env = jinja2.Environment(loader=jinja2.FileSystemLoader('templates/'), autoescape=False) |
jinja2_loader = Jinja2Loader(jinja2_env) |
settings = dict(template_loader=jinja2_loader) |
class grant_sql(tornado.web.RequestHandler): |
def get(self, database_name): |
template = jinja2_env.get_template("index.html") |
text1 = '<h1>権限付与SQL作成</h1>\n' |
text1 += '<form method="post" action="../result/">\n' |
try : |
database_dsn = "{}.fdb".format(database_name) |
co = fdb.connect(dsn=database_dsn, user='eagle-eight', password='masterkey', charset='UTF8') |
cur = co.cursor() |
text1 += '<h2>権限付与対象ユーザ</h2>\n' |
SQL = "select sec$user_name from sec$users where sec$admin = false" |
cur.execute(SQL) |
result = cur.fetchall() |
if(len(result)>0) : |
text1 += '<div class="result">\n' |
for r in result : |
user_mname = r[0].rstrip() |
text1 += '<label><input type="checkbox" name="user_name" value="{}">{}</label><br>\n'.format(user_mname, user_mname) |
text1 += '</div>\n' |
text1 += '<h2>権限</h2>\n' |
text1 += '<h3>テーブル</h3>\n' |
SQL = "select rdb$relation_name from rdb$relations where (rdb$system_flag is null or rdb$system_flag = 0) and rdb$view_blr is null" |
cur.execute(SQL) |
result = cur.fetchall() |
if(len(result)>0) : |
text1 += '<div class="result">\n<table class="table_type1">\n' |
for r in result : |
table_name = r[0].rstrip() |
text1 += '<td class="td_column1">{}</td><td class="td_column2"><label><input type="checkbox" name="table_all" value="{}">ALL</label><br><label><input type="checkbox" name="table_select" value="{}">SELECT</label></td><tr>'.format(table_name, table_name, table_name) |
text1 += '</table>\n</div>\n' |
text1 += '<h3>ビュー</h3>\n' |
SQL = "select rdb$relation_name from rdb$relations where (rdb$system_flag is null or rdb$system_flag = 0) and rdb$view_blr is not null" |
cur.execute(SQL) |
result = cur.fetchall() |
if(len(result)>0) : |
text1 += '<div class="result">\n<table class="table_type1">\n' |
for r in result : |
view_name = r[0].rstrip() |
text1 += '<td class="td_column1">{}</td><td class="td_column2"><label><input type="checkbox" name="view_select" value="{}">SELECT</label></td><tr>'.format(view_name, view_name) |
text1 += '</table>\n</div>\n' |
text1 += '<h3>シーケンス</h3>\n' |
SQL = "select rdb$generator_name from rdb$generators where rdb$system_flag = 0" |
cur.execute(SQL) |
result = cur.fetchall() |
if(len(result)>0) : |
text1 += '<div class="result">\n<table class="table_type1">\n' |
for r in result : |
sequence_name = r[0].rstrip() |
text1 += '<td class="td_column1">{}</td><td class="td_column2"><label><input type="checkbox" name="sequence_usage" value="{}">USAGE</label></td><tr>'.format(sequence_name, sequence_name) |
text1 += '</table>\n</div>\n' |
text1 += '<h3>プロシージャ</h3>\n' |
SQL ="select rdb$procedure_name from rdb$procedures where rdb$system_flag = 0" |
cur.execute(SQL) |
result = cur.fetchall() |
if(len(result)>0) : |
text1 += '<div class="result">\n<table class="table_type1">\n' |
for r in result : |
procedure_name = r[0].rstrip() |
text1 += '<td class="td_column1">{}</td><td class="td_column2"><label><input type="checkbox" name="procedure_execute" value="{}">EXECUTE</label></td><tr>'.format(procedure_name, procedure_name) |
text1 += '</table>\n</div>\n' |
except : |
traceback.print_exc() |
text1 += '<input type="image" src="http://192.168.25.38/image/poligon_gold-olive_7.png">\n' |
text1 += '</form>\n' |
self.write(template.render(title="権限付与SQL作成", render_text=text1)) |
class sql_result(tornado.web.RequestHandler): |
def post(self) : |
template = jinja2_env.get_template("index.html") |
text1 = '<h1>権限付与SQL作成</h1>\n' |
user_name_list = self.get_arguments("user_name") |
table_all_list = self.get_arguments("table_all") |
table_select_list = self.get_arguments("table_select") |
view_select_list = self.get_arguments("view_select") |
sequence_usage_list = self.get_arguments("sequence_usage") |
procedure_execute_list = self.get_arguments("procedure_execute") |
if len(user_name_list) > 0 : |
text1 += '<div class="result">\n' |
for user_name in user_name_list : |
text1 += '/* {}ユーザへの権限の付与 */<br>\n'.format(user_name) |
for table_all in table_all_list : |
text1 += 'grant all on {} to {};<br>\n'.format(table_all, user_name) |
for table_select in table_select_list : |
text1 += 'grant select on {} to {};<br>\n'.format(table_select, user_name) |
for view_select in view_select_list : |
text1 += 'grant select on {} to {};<br>\n'.format(view_select, user_name) |
for sequence_name in sequence_usage_list : |
text1 += 'grant usage on sequence {} to {};<br>\n'.format(sequence_name, user_name) |
for procedure_name in procedure_execute_list : |
text1 += 'grant execute on procedure {} to {};<br>\n'.format(procedure_name, user_name) |
text1 += '</div>\n' |
self.write(template.render(title="権限付与SQL作成", render_text=text1)) |
def make_app(): |
return tornado.web.Application([ |
(r"/grant_sql/result/", sql_result), |
(r"/grant_sql/(.*)/", grant_sql), |
], |
#debug=True, |
autoreload=True |
) |
def main(): |
app = make_app() |
app.listen(8888) |
IOLoop.current().start() |
if __name__ == '__main__': |
main() |
1 |
2 |
3 |
4 |
5 |
6 |
7 |
8 |
9 |
10 |
11 |
12 |
13 |
14 |
15 |
16 |
17 |
18 |
19 |
20 |
21 |
22 |
23 |
24 |
25 |
26 |
27 |
28 |
29 |
30 |
31 |
32 |
33 |
34 |
35 |
36 |
37 |
38 |
39 |
40 |
41 |
42 |
43 |
44 |
45 |
46 |
47 |
48 |
49 |
50 |
51 |
52 |
53 |
54 |
55 |
56 |
57 |
58 |
59 |
60 |
61 |
62 |
63 |
64 |
65 |
66 |
67 |
68 |
69 |
70 |
71 |
72 |
73 |
74 |
75 |
76 |
77 |
78 |
79 |
80 |
81 |
82 |
83 |
84 |
85 |
86 |
87 |
88 |
89 |
90 |
91 |
92 |
93 |
94 |
95 |
96 |
97 |
98 |
99 |
100 |
101 |
102 |
103 |
104 |
105 |
106 |
107 |
108 |
109 |
110 |
111 |
112 |
113 |
114 |
115 |
116 |
117 |
118 |
119 |
120 |
121 |
122 |
123 |
124 |
125 |
126 |
127 |
128 |
129 |
130 |
131 |
132 |
133 |
134 |
135 |
136 |
137 |
138 |
139 |
140 |
141 |
以下の内容で templates/layout.html を作成する。
<!DOCTYPE html> |
<html lang="ja"> |
<head> |
<meta charset="UTF-8"> |
<title>{{title}}</title> |
<style> |
body { background-color: black; color: white; margin: 30px; } |
h1 { color: gold; border-bottom: double 3px; font-weight: normal; } |
h2 { color: navajowhite; border-bottom: solid 2px; border-left: solid 20px; padding: 3px; font-weight: normal; } |
h3 { border-bottom: solid 1px white; padding: 3px; font-weight: normal; } |
h4 { border-top: solid 1px gold; padding: 3px; margin-top: 30px; font-weight: normal; } |
h4 p { padding: 0px; margin: 0px; } |
.left { text-align: left; } |
.center { text-align: center; } |
.right { text-align: right; } |
.img_overlay { position: absolute; left: 0; top: 0; right: 0; bottom: 0; margin: 0; background: rgba(100, 100, 100, 0.9); z-index: 2147483640; } |
.result { margin-top: 10px; margin-bottom: 10px; margin-left: 60px; margin-right: 60px; } |
a { color: deepskyblue; text-decoration: underline dotted lightskyblue; } |
.img_coverart { margin-top: 0px; margin-bottom: 3px; margin-left: 0px; margin-right: 6px; } |
table { color: white; padding: 0px; margin: 0px; border-collapse: collapse; } |
.table_default { width: 100%; border: none; } |
.th_default { border-bottom: solid 1px white; padding-top: 3px; padding-left: 10px; padding-right: 10px; padding-bottom: 3px; text-align: left; } |
.td_default { border-bottom: solid 1px white; padding-top: 3px; padding-left: 10px; padding-right: 10px; padding-bottom: 3px; } |
.td_column1 { width: 300px; padding: 6px; vertical-align: top; } |
.td_column2 { width: 300px; padding: 6px; border-left: solid 1px white; } |
input[type="text"] { padding: 3px 3px; font-size: 16px; margin-left: 20px; margin-top: 4px; margin-right: 0px; margin-bottom: 8px ; } |
input[type="checkbox"] { margin: 6px; font-size: 16px; } |
input[type="image"] { margin-left: 0px; margin-top:04px; margin-right: 0px; margin-bottom: 0px ; } |
.text_movie_title { width: 400px; } |
.text_movie_caption { width: 800px; } |
</style> |
</head> |
<body> |
{% block body %}{% endblock %} |
</body> |
</html> |
1 |
2 |
3 |
4 |
5 |
6 |
7 |
8 |
9 |
10 |
11 |
12 |
13 |
14 |
15 |
16 |
17 |
18 |
19 |
20 |
21 |
22 |
23 |
24 |
25 |
26 |
27 |
28 |
29 |
30 |
31 |
32 |
33 |
34 |
35 |
36 |
以下の内容で templates/index.html を作成する。
{% extends "layout.html" %} |
{% block body %} |
{{render_text}} |
<footer><h4><p class="right">Powered by Tornado 6.1</p><p class="right">copyright 2022 eagle eight</p></h4></footer> |
{% endblock %} |
1 |
2 |
3 |
4 |
5 |
コマンドプロンプトで python app_grant_sql.py を実行する。
http://localhost:8888/<Firebirdデータベースの拡張子ナシのファイル名>/ にアクセスして動作を確認する。